The XML library is a library for processing XML documents written in XML format.
The outline of the XML library is as follows.
Generate XML objects from XML documents
The XML_CreateXmlObject function parses an XML document, transforms it into an XML object, and returns an XML handle to reference this object.
The XML_CreateXmlObject function transforms each element of the XML document into a node represented by an XML_Node structure and links it to the XML object. Each node constitutes a single interconnected graph starting from the root node.
There are three types of node links represented by the XML_Node structure: links to the next node (sibling node) in the same hierarchy, links to the child node, and links to the parent node (not shown in the figure below). Each function in the XML library uses these links to perform processing.
If the element has attributes, the attribute name and value are stored in the XML_Attribute structure and linked to the node's XML_Node structure. If there are multiple attributes, the second and subsequent attributes are linked from the XML_Attribute structure of the previous attribute.
Most functions in the XML library take a XML handle as a parameter and process the XML object referenced by this handle.
Example: A graph of nodes
An XML document:
<request_message> <issuer>SALES0001</issuer> <ship_order> <date>2021/09/10</date> <customer> <customerID>C00001</customerID> <name>A corporation</name> </customer> <orders> <order> <productID>PID0000100</productID> <name>A product</name> </order> <order> <productID>PID0000200</productID> <name>B product</name> </order> </orders> </ship_order> </request_message>
A graph of nodes: Each node is represented by an XML_Node structure.
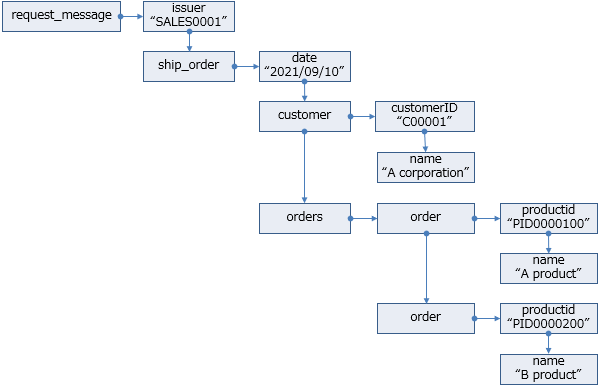
Example: A graph of attributes
An XML document:
<message>
<email msgID='10010' from='a@b.c'>Good!</email>
</message>
A graph of attributes: Each attribute is represented by an XML_Attribute structure.
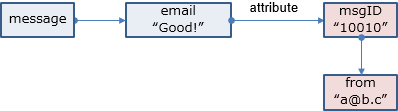
Dump contents of XML object
For debugging, use the following function to dump the contents of an XML object into a log message.
- XML_DumpNode function
- Dumps the contents of the node into log messages.
- XML_DumpXmlObject function
- Dumps the contents of the entire XML object with log messages.
Convert XML objects into XML documents
Use the following function to convert the contents of an XML object into an XML document and output it.
- XML_SaveAsXmlFile function
- Converts the contents of the specified XML object to a UTF-8 XML document and saves it to a file.
- XML_SaveAsXmlMemory function
- Converts the contents of the specified XML object to a UTF-8 XML document and outputs it to memory.
These functions do not support output in encodings other than UTF-8.
Release the XML object
Whenever you no longer need an XML object, call the XML_ReleaseXmlObject function to free the XML object's resources.
How to use the node path
You can use the node path to easily specify each node linked to the XML object.
The node path is a character string in which node names are concatenated in order with '/' from the upper level to the lower level. For example, write as follows.
"ship_order/customer/name"
Specify a full path
A full path is a kind of the node path . You can specify a node with a full path that describes all the hierarchies from directly under the root node.
For example, if you specify "ship_order/customer/name" in the following example, you can specify the "name" node in the figure below.
<request_message> ← root node <issuer>SALES0001</issuer> <ship_order> <date>2021/09/10</date> <customer> <customerID>C00001</customerID> <name>A corporation</name> ← Specifies this node </customer> </ship_order> </request_message>
You can specify the full path with the following function.
Specify a relative path
A relative path is a kind of the node path. You can specify a node by specifying the parent node and the relative path that describes only the hierarchy below the parent node.
For example, if you specify the "ship_order" node as the parent node and specify "customer / customerID" as the relative path in the following example, you can specify the "customerID" node in the figure below.
<request_message> <issuer>SALES0001</issuer> <ship_order> ← Specify this node as the parent node <date>2021/09/10</date> <customer> <customerID>C00001</customerID> ← Specifies this node by the relative path <name>A corporation</name> </customer> </ship_order> </request_message>
You can specify the relative path with the following function.
XML_GetChildNodeValueByPath function
XML_SetChildNodeValueByPath function
Use wildcards (*)
You can specify * on the last node in the node path to match the first node in the hierarchy. For example, write as follows.
"*"
"ship_order/*"
"ship_order/customer/*"
You can get the "customerID" node by specifying "ship_order/customer/*" in the following example.
<request_message> ← root node <issuer>SALES0001</issuer> <ship_order> <date>2021/09/10</date> <customer> <customerID>C00001</customerID> ← You can get this node. <name>A corporation</name> </customer> </ship_order> </request_message>
You can specify wildcards (*) with the following function.
Note on uniqueness of the node path
In XML, multiple elements (nodes) with the same name can be placed in the same hierarchy. However, when using a node path to specify a node, be aware that if the node specified in the node path cannot be uniquely identified, an error may occur and the function may fail.
When using the following functions, all higher intermediate nodes other than the last node in the node path must be uniquely identified. If the intermediate nodes cannot be uniquely identified, the function will fail and return XML_NOT_UNIQUE_INTERMEDIATE_NODE error.
For example, in the following example, if you specify "ship_order/orders/order/name" with the full path, the "order" of the intermediate node cannot be uniquely identified, resulting in XML_NOT_UNIQUE_INTERMEDIATE_NODE error.
<request_message> ← root node <issuer>SALES0001</issuer> <ship_order> <orders> <order> ← "order" node is not unique. <productID>PID0000100</productID> <name>A product</name> </order> <order> ← "order" node is not unique. <productID>PID0000200</productID> <name>B product</name> </order> </orders> </ship_order> </request_message>
To access such data that has intermediate nodes that cannot be uniquely identified using a node path, first search for a node by specifying a node path that can uniquely identify all upper intermediate nodes other than the last node. Next, specify the obtained node to access the next node (sibling node) or child node.
For example, in the following example, if you specify "ship_order/orders/order", you can get the first "order" node by calling the XML_FindNodeByPath function with the full path. Pass this node to the XML_NextNode function to get the next node (sibling node), or specify this node as the parent node and use a relative path to access the child nodes.
<request_message> ← root node <issuer>SALES0001</issuer> <ship_order> <date>2021/09/10</date> <customer> <customerID>C00001</customerID> <name>A corporation</name> </customer> <orders> <order> ← Search result <productID>PID0000100</productID> ← child node <name>A product</name> ← child node </order> <order> ← next node (sibling node) <productID>PID0000200</productID> <name>B product</name> </order> </orders> </ship_order> </request_message>
When using the following functions, nodes in all hierarchies of the node path must be uniquely identifiable. If the intermediate node cannot be uniquely identified, XML_NOT_UNIQUE_INTERMEDIATE_NODE error will occur as above, and if the last node cannot be uniquely identified, XML_NOT_UNIQUE_NODE error will occur and the function will fail.
XML_SetNodeValueByPath function
XML_GetChildNodeValueByPath function
XML_SetChildNodeValueByPath function
For example, in the following example, if you specify "ship_order/comments/comment" with the full path, the "comment" of the last node cannot be uniquely identified, resulting in XML_NOT_UNIQUE_NODE error.
<request_message> ← root node <issuer>SALES0001</issuer> <ship_order> <comments> <comment>Sample</comment> ← "comment" node is not unique. <comment>Opticon</comment> ← "comment" node is not unique. </comments> <orders> </orders> </ship_order> </request_message>
Get the root node information
Use the following function to get information about the root node.- XML_GetRootNode function
- Get the root node.
- XML_GetRootNodeName function
- Gets the name of the root node.
Search for a node
Use the following function to search for a node by specifying a node path. These functions also allow you to specify wildcards (*) in the node path.- XML_FindNodeByPath function
- Search for a node by specifying a full path.
- XML_FindChildNodeByPath function
- Search for a node by specifying a parent node and a relative path.
If you have a pointer to the node's XML_Node structure, you can follow the node's links directly using the following function.
- XML_NextNode function
- Gets the next node (sibling node).
- XML_ParentNode function
- Gets the parent node.
- XML_FindChildNodeByPath function
- You can get the first child node of the specified node by specifying "*" for childNodePath.
Get the value of a node
Use the following function to get the value of a node specified by a node path.- XML_GetNodeValueByPath function
- Gets the value of a node specified by a full path.
- XML_GetChildNodeValueByPath function
- Gets the value of a node specified by a parent node and a relative path.
- XML_GetNodeValue function
- Gets the value of the specified node.
Set the value of a node
Use the following function to set the value of the node specified by a node path.- XML_SetNodeValueByPath function
- Sets the value of a node specified by a full path.
- XML_SetChildNodeValueByPath function
- Sets the value of the node specified by the parent node and the relative path.
If you have a pointer to the node's XML_Node structure, you can set the value of the node using the following function.
- XML_SetNodeValue function
- Sets the value of the specified node.
Add a node
Use the following function to add a node.
- XML_AddChildNode function
- Adds a child node to the specified node.
- XML_SetNodeValueByPath function
- Sets the value of the node specified by a full path. If the specified node at the end of the full path does not exist , it will be added automatically.
- XML_SetChildNodeValueByPath function
- Sets the value of the node specified by a parent node and the relative path. If the specified node at the end of the relative path does not exist , it will be added automatically.
Delete a node
Use the following function to delete a node.- XML_DeleteNode function
- Deletes the specified node. If the specified node has child nodes, they can be deleted at the same time.
Get node attributes
Use the following function to get the XML_Attribute structure of the node's attributes.- XML_FindAttribute function
- Search for a node attribute by name.
- XML_GetAttribute function
- Gets the XML_Attribute structure for the first attribute linked to the node.
- XML_NextAttribute function
- If the following attribute is linked to the specified attribute, the XML_Attribute structure of that attribute is acquired.
The name and value of an attribute are taken from the name and value members of the XML_Attribute structure.
Set node attributes
Use the following function to set attributes on the node.- XML_SetAttribute function
- Sets an attribute for the specified node.
Delete node attributes
Use the following function to delete attributes on the node.- XML_DeleteAttributes function
- Deletes attributes of the specified node.
Last updated: 2021/10/21