Syntax
bool AM_EnableTextEdit(
MENU_HANDLE menu,
int id
);
Parameters
- menu
- [in] Menu handle
- id
-
[in] Item ID of menu item.
Return value
Returns true if the function succeeds, false otherwise.
Returns false if the menu item specified by id is not an input field.
Returns false if the menu item specified by id is not an input field.
Remarks
Enables the input / edit operation of the input field specified by id.This function is used to programmatically enable input / edit operations on the input field for which the AM_EDIT_DISABLE_TEXT_EDIT option was specified in the EditOption of the AM_EditParam structure to disable input / edit operations.
Specify the AM_EDIT_DISABLE_TEXT_EDIT option in the input field where you want to allow key input only under specific conditions, and call this function when you start input.
To disable the input / edit operation of the input field programmatically, call the AM_DisableTextEdit function.
Use the AM_IsTextEditEnabled function to check if the input / edit operation of the input field is enebled.
Requirements
Header file:
lib.hLibrary file:
AdvancedMenu.h (Ver. 1.6.1 or later)
libAdvancedMenu.a (Ver. 1.6.1 or later)
libSTARTUPOPH5000.a
Sample
There are two input fields on the screen, and in the initial state, you can read the barcode with the [SCAN] key.When you press a character key, it switches to the character input mode, and when you press the [ENT] key, it returns to the barcode reading mode again.
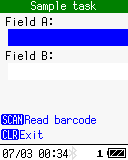
You can move the focus to the previous or next input field by pressing the [Q1] and [Q2] keys.
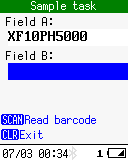
When you press the [SCAN] key and read the barcode, the focus automatically advances to the next field.
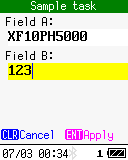
Press a number key to switch to character input mode. You can move the cursor left or right in the input field by pressing the [Q1] and [Q2] keys in the character input mode.
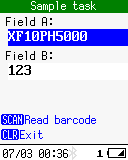
Press the [ENT] key to confirm your input and the focus will automatically advance to the next field.
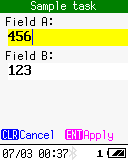
Press a number key to clear the current data and switch to character input mode.
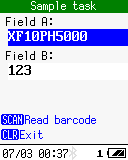
Press the [CLEAR] key to cancel the character input and restore the original data.
#include <stdio.h>
#include <string.h>
#include "lib.h"
#include "AdvancedMenu.h"
// Prototypes
void task(void);
///////////////////////////////////////////////////////////////////
// Color palette
//////////////////////////////////////////////////////////
#define FORE_COLOR RGB_WHITTE
#define BACK_COLOR 0xf7f7f7 //Light gray
#define SELECT_COLOR RGB_BLUE
#define DISABLE_COLOR 0xb0b0b0 //Gray
#define GIUDE_BUTTON RGB_BLUE
#define APPLY_COLOR RGB_MAGENTA
#define TITLE_TASK_COLOR 0x008000 //Dark Green
static const AM_ColorPalette CustomColor[] = {
//ForeColor, BackColor, Select_ForeColor, Select_BackColor, Control_ForeColor
{ RGB_BLACK, BACK_COLOR, RGB_WHITE, SELECT_COLOR, GIUDE_BUTTON }, //#0: Base color
{ GIUDE_BUTTON, BACK_COLOR, 0, 0, GIUDE_BUTTON }, //#1: Guide color
{ RGB_WHITE, TITLE_TASK_COLOR, 0, 0, 0 }, //#2: Title 0f tasks
{ RGB_BLACK, RGB_WHITE, RGB_WHITE, RGB_BLUE, RGB_YELLOW }, //#3: Input field color
{ RGB_BLACK, RGB_WHITE, RGB_BLACK, RGB_YELLOW, RGB_BLUE }, //#4: Input editing color
{ APPLY_COLOR, BACK_COLOR, 0, 0, APPLY_COLOR }, //#5: Apply color
};
#define PX_BASE 0 // Base color
#define PX_GUIDE 1 // Guide color
#define PX_TITLE 2 // Title color
#define PX_INPUT 3 // Input field color
#define PX_EDIT 4 // Input editing color
#define PX_APPLY 5 // Apply color
//////////////////////////////////////////////////////////
// Options for task screen
//////////////////////////////////////////////////////////
static const AM_Option TaskOption = {
RGB_BLACK, // MenuForeColor
DISABLE_COLOR, // MenuDisabledForeColor
BACK_COLOR, // MenuBackColor
sizeof(CustomColor)/sizeof(AM_ColorPalette), // NumberOfColorPalette
(pAM_ColorPalette)CustomColor, // ColorPalettes
0, // OptionFlag
MEDIUM_FONT, // DefaultFont
1 // DefaultLineSpacing
};
//////////////////////////////////////////////////////////
// Text field parameter
//////////////////////////////////////////////////////////
static char EditBuf_A[15+1] = {""};
static const AM_EditParam EditParam_A = {
15, // MaxDigits
RGB_WHITE, // Cursor foregraund Color
RGB_BLUE, // Cursor backgraund Color
RGB_WHITE, // Shift mode foregraund Color
RGB_BLUE, // Shift mode backgraund Color
AM_EDIT_DISABLE_TEXT_EDIT | AM_EDIT_MOVE_CURSOR_BY_Q1Q2 | AM_EDIT_DISALLOW_ALPHA, // EditOption
NULL, // alphaCandidateTable
EditBuf_A, // Value
sizeof(EditBuf_A) // ValueBufSize
};
static char EditBuf_B[15+1] = {""};
static const AM_EditParam EditParam_B = {
15, // MaxDigits
RGB_WHITE, // Cursor foregraund Color
RGB_BLUE, // Cursor backgraund Color
RGB_WHITE, // Shift mode foregraund Color
RGB_BLUE, // Shift mode backgraund Color
AM_EDIT_DISABLE_TEXT_EDIT | AM_EDIT_MOVE_CURSOR_BY_Q1Q2 | AM_EDIT_DISALLOW_ALPHA, // EditOption
NULL, // alphaCandidateTable
EditBuf_B, // Value
sizeof(EditBuf_B) // ValueBufSize
};
static char prev_EditBuf[15+1]; //for saving previous value.
//////////////////////////////////////////////////////////
// Definition of the menu items
//////////////////////////////////////////////////////////
// Item ID
enum _TASK_ITEM_ID {
TASK_ID_FIELD_A = 1,
TASK_ID_FIELD_B,
TASK_ID_SCAN,
TASK_ID_EXIT,
TASK_ID_CANCEL,
TASK_ID_APPLY,
};
// Table of the menu items
static const AM_MenuItem TaskMenuTable[] = {
// itemID, y, x, menuText, Palette, visible, enabled, selectable, showControl, checked, font
{0, 0, 0, "Sample task", PX_TITLE, true, true, false, AM_TITLE_LINE, false, MEDIUM_FONT},
{0, AM_PIX(16), 1, "Field A:", PX_BASE, true, true, false, AM_NO_CONTROL},
{TASK_ID_FIELD_A, AM_PIX(29), 1, (void *)&EditParam_A, PX_INPUT, true, true, true, AM_TEXT_EDIT, false, LARGE_FONT},
{0, AM_PIX(50), 1, "Field B:", PX_BASE, true, true, false, AM_NO_CONTROL},
{TASK_ID_FIELD_B, AM_PIX(63), 1, (void *)&EditParam_B, PX_INPUT, true, true, true, AM_TEXT_EDIT, false, LARGE_FONT},
{TASK_ID_SCAN, AM_PIX(114), 0, "Read barcode", PX_GUIDE, true, true, false, AM_SCAN_ICON},
{TASK_ID_EXIT, AM_PIX(129), 0, "Exit", PX_GUIDE, true, true, false, AM_CLEAR_ICON},
{TASK_ID_CANCEL, AM_PIX(129), 0, "Cancel", PX_GUIDE, false, true, false, AM_CLEAR_ICON},
{TASK_ID_APPLY, AM_PIX(129), AM_PIX(64), "Apply", PX_APPLY, false, true, false, AM_ENT_ICON},
{-1}
};
//////////////////////////////////////////////////////////
// main
//////////////////////////////////////////////////////////
void main(void)
{
while(1){
printf("\fPress ENT to start");
while(1){
if (kbhit()){
if (getchar() == ENT_KEY){
task();
break;
}
}
Idle();
}
}
}
//////////////////////////////////////////////////////////
// task
//////////////////////////////////////////////////////////
void task(void)
{
bool nowEditing = false;
MENU_HANDLE hMenu;
int event;
char barcodebuff[32];
AM_BarcodeBuffer buffer;
int selectedLine;
memset(EditBuf_A,0,sizeof(EditBuf_A));
memset(EditBuf_B,0,sizeof(EditBuf_A));
hMenu = AM_CreateMenu(TaskMenuTable, (const pAM_Option)&TaskOption);
buffer.dataBuf = barcodebuff;
buffer.dataBufLength = sizeof(barcodebuff);
// Set option commands
// B0: Disable all symbology
// B2: Enable CODE39
// R4: Enable EAN
AM_ConfigBarcodeReader(hMenu, &buffer, "B0B2R4");
AM_ShowMenu(hMenu, AM_SELECT_ANY_ID);
AM_EnableBarcodeReader(hMenu);
while(1){
event = AM_ExecMenu(hMenu);
selectedLine = AM_GetSelectedLine(hMenu);
if (!nowEditing){
//// Scan mode ////////////////////////////
if (event == AMENU_BARCODE_READ){
//Get barcode
AM_DisableBarcodeReader(hMenu);
AM_SetText(hMenu, selectedLine, barcodebuff);
//Skip to the next field
if (selectedLine == TASK_ID_FIELD_A){
AM_SelectLine(hMenu, TASK_ID_FIELD_B);
}else if(selectedLine == TASK_ID_FIELD_B){
AM_SelectLine(hMenu, TASK_ID_FIELD_A);
}
AM_EnableBarcodeReader(hMenu);
}else if((event >='0' && event<='9') || event == SHIFT_KEY){
// Switching to the text edit mode
AM_DisableBarcodeReader(hMenu);
//Save current value for cancel
strcpy(prev_EditBuf, AM_GetText(hMenu,selectedLine ));
// Clear the text field
AM_SetText(hMenu, selectedLine, "");
// Push back the last key code
ungetc(event, stdin);
// Enable text edit
AM_EnableTextEdit(hMenu, selectedLine);
AM_SetPaletteIndex(hMenu, selectedLine, PX_EDIT);
AM_SetVisibleRange(hMenu, TASK_ID_SCAN, TASK_ID_EXIT, false);
AM_SetVisibleRange(hMenu, TASK_ID_CANCEL, TASK_ID_APPLY, true);
nowEditing=true;
}else if (event == CLR_KEY){
//Exit
break;
}
}else{
//// Text edit mode ///////////////////////
if (event == CLR_KEY || event == ENT_KEY){
// Disable text edit
AM_DisableTextEdit(hMenu, selectedLine);
AM_SetPaletteIndex(hMenu, selectedLine, PX_INPUT);
if (event == CLR_KEY){
//Restore previous value
AM_SetText(hMenu, selectedLine, prev_EditBuf);
}else{
//ENT_KEY
//...
//Skip to the next field
if (selectedLine == TASK_ID_FIELD_A){
AM_SelectLine(hMenu, TASK_ID_FIELD_B);
}else if(selectedLine == TASK_ID_FIELD_B){
AM_SelectLine(hMenu, TASK_ID_FIELD_A);
}
}
// Back to scan mode
AM_EnableBarcodeReader(hMenu);
AM_SetVisibleRange(hMenu, TASK_ID_CANCEL, TASK_ID_APPLY, false);
AM_SetVisibleRange(hMenu, TASK_ID_SCAN, TASK_ID_EXIT, true);
nowEditing=false;
}
}
}
AM_DisableBarcodeReader(hMenu);
AM_ReleaseMenu(hMenu);
return;
}
See also
AM_DisableTextEdit function
Last updated: 2021/07/03